Game Loops Explained: Real-Time Rendering Basics
- abekesora
- Apr 17
- 6 min read
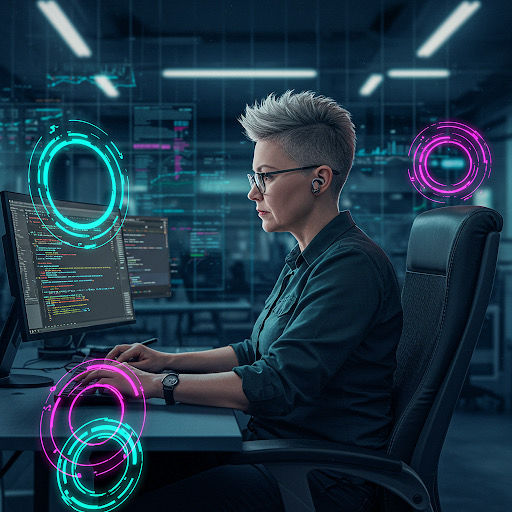
Looping In: Why Game Loops Matter
Every real‑time application—from the simplest arcade clone to the latest AAA open‑world epic—is driven by a game loop: a continuous cycle that polls input, updates game state, and renders frames to the screen. Without this heartbeat, the world would stand still—no movement, no AI, no physics simulation. The game loop provides the temporal framework that ties together all subsystems into a coherent interactive experience, ensuring that player actions produce timely responses and that the simulation marches forward at a consistent cadence.
Beyond mere functionality, the design of the game loop profoundly influences responsiveness, performance, and determinism. A well‑engineered loop delivers smooth frame rates, precise input latency, and reliable synchronization between subsystems (physics, audio, networking). It also establishes the foundation for important techniques—fixed‑timestep physics, interpolation, smooth animation blending—that together create the illusion of a living, breathing world. In short, the game loop is not just boilerplate code; it is the beating heart of any interactive simulation.
Anatomy of a Basic Game Loop
A typical real‑time rendering loop can be broken down into five core phases:
1. Initialization
2. Input Handling
3. State Update
4. Rendering
5. Cleanup/Termination
6. Initialization
Before entering the loop, the engine allocates resources: loads textures and meshes, compiles shaders, sets up audio buffers, and initializes subsystems like physics and networking. Proper initialization ensures that once the loop begins, no costly disk or GPU stalls disrupt frame pacing.
7. Input Handling
At the start of each iteration, the loop polls input devices—keyboard, mouse, gamepad—or reads OS events. This phase typically involves draining event queues and updating input state (e.g., which keys are pressed). Capturing input early in the loop guarantees that player commands affect the very next update cycle.
8. State Update
With fresh inputs, the engine advances game logic: moves characters, simulates physics, executes AI routines, and processes game rules (collisions, health, scores). This is where world state changes occur—positions, velocities, animations, and game variables are all recomputed based on elapsed time and player actions.
9. Rendering
Once the state is updated, the renderer constructs a new frame: culling invisible objects, issuing draw calls, and compositing post‑processing effects. Finally, the loop swaps front and back buffers (or presents the frame), making the newly rendered image visible to the player.
10. Cleanup/Termination
The loop repeats until a termination condition is met—player quits, crashes, or finishes a level. After exiting the cycle, the engine releases resources, writes out save data, and shuts down subsystems gracefully, leaving no memory or handle leaks.
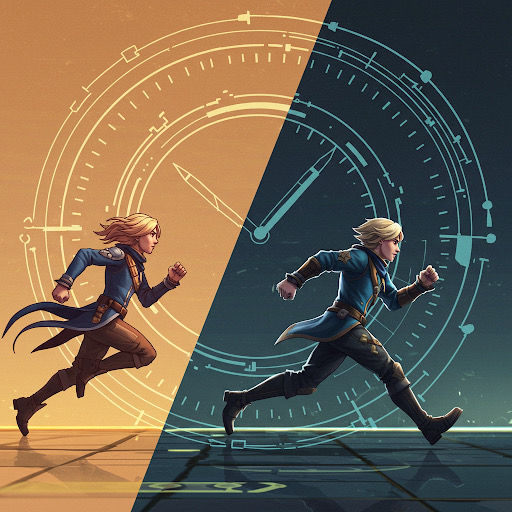
Delta Time and Frame Rates Explained
1. What Is Delta Time?
Delta time (Δt) is the elapsed time between two successive frames, usually expressed in seconds or milliseconds. Instead of moving objects by a fixed amount per frame—which would vary wildly with frame rate—engines multiply velocities and animations by Δt to achieve frame‑rate–independent motion.
2. Why Frame Independence Matters
On a high‑end PC running at 144 FPS, a character moving 300 units/second should shift roughly 2.08 units per frame. On a low‑end laptop at 30 FPS, that same character must move 10 units per frame. By scaling movement and physics by Δt, developers ensure that gameplay remains consistent regardless of hardware performance.
3. Calculating Delta Time
Each loop iteration records the current timestamp (e.g., via a high‑resolution timer) and subtracts the previous frame’s timestamp. The result is Δt.
Pseudocode:
// Start of loop logic
lastTime = currentTime();
while (running) {
→ current = currentTime();
→ deltaTime = current - lastTime;
→ lastTime = current;
→ update(deltaTime);
→ render();
}
// End of loop logic
This simple pattern underpins nearly every real‑time engine.
4. Pitfalls of Variable Δt
When Δt spikes—due to a hitch, garbage collection, or I/O stall—updating with a large Δt can cause objects to tunnel through geometry, break collision stability, or produce jarring animation jumps. Conversely, very small Δt values can amplify numerical precision errors. Developers often clamp Δt to a maximum value (e.g., 0.05 s) to mitigate these issues.
5. Smoothing and Frame Rate Metrics
To provide feedback and smoothing, engines compute moving averages of Δt (or instantaneous FPS = 1/Δt) and display them in debug overlays. Some systems also implement exponential smoothing on how Δt affects motion, creating a more visually consistent experience even when frame times fluctuate.
Fixed vs Variable Update Methods
Game loops must decide how often to update simulation logic relative to rendering frames. Fixed‐timestep updates advance the world by a constant Δt each cycle—commonly 16.67 ms for 60 Hz—ensuring deterministic behavior. Physics engines particularly benefit: collisions resolve consistently, and multiplayer lockstep can synchronize across machines. However, if a frame takes longer than the fixed interval, the loop may perform multiple back‐to‐back updates (“catch‑up”), potentially causing input lag or CPU spikes.
Variable‐timestep updates, in contrast, pass the actual Δt between frames directly into update routines. This approach maximizes responsiveness on high‑end hardware—if you run at 120 FPS, your game feels twice as smooth—and avoids catch‑up storms on slow frames. Yet without caps or clamping, large Δt spikes (e.g., during a hitch) can destabilize physics and break gameplay. Many developers therefore implement Δt clamping (e.g., max 50 ms) to prevent runaway updates.
A hybrid approach blends both methods: core systems (physics, AI) run on a fixed timer, while noncritical logic (particle effects, background animations) uses variable Δt. Between fixed updates, the engine can interpolate object states for rendering, smoothing motion without compromising determinism. This decoupling allows the game to maintain a stable simulation backbone while leveraging extra CPU time for visual fidelity.
Designers must also consider frame‐rate independence. Fixed updates require catch‑up logic and interpolation, whereas pure variable updates demand robust clamping and robustness checks. Both systems need safeguards—excessive fixed‐step loops or overly large Δt values can lead to spiraling performance issues. Profiling and telemetry help tune iteration budgets, ensuring the loop neither starves nor overwhelms the CPU.
Ultimately, the choice hinges on project requirements. Competitive multiplayer and precise physics simulations lean toward fixed timesteps for predictability. Fast‑paced action titles and cinematic experiences often favor variable updates for fluidity. By understanding the trade‑offs and potentially combining methods, developers craft loops that balance stability, responsiveness, and performance.
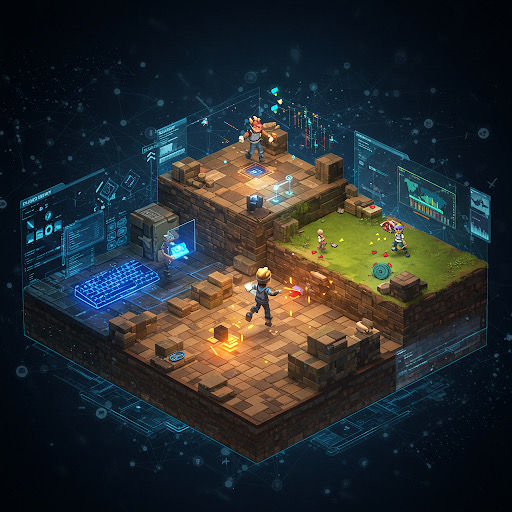
Handling Input, Physics, and Rendering
The ordering of subsystems within the loop critically affects responsiveness and consistency. Input handling typically occurs first, polling hardware states or draining OS event queues so that player actions influence the very next simulation step. Some engines batch inputs into a ring buffer, allowing rewinding or replay for rollback networking—essential in fighting games or lockstep architectures.
Next comes the physics update. Using either fixed or variable timesteps, the loop advances rigid bodies, computes collisions, and resolves constraints. Engines often subdivide this phase into broad‐phase (spatial partitioning) and narrow‐phase (precise intersection tests) stages for efficiency. Deterministic physics relies on consistent timesteps; when mixing update methods, interpolation buffers smooth out object positions between discrete physics ticks.
Following physics, game logic and AI apply—character controllers, state machines, and behavior trees react to the updated world state. By separating logic from physics, developers can optimize subsystems independently: AI can run at lower frequencies or on separate threads, while time‐critical physics maintains real‐time fidelity.
Finally, the rendering phase begins. The engine culls unseen objects (frustum, occlusion), issues draw calls (batching where possible), and executes post‐processing shaders. Double or triple buffering ensures smooth presentation, while vsync or adaptive sync technologies mitigate tearing. Some advanced engines decouple rendering onto a separate thread or GPU queue to overlap computation and drawing, squeezing maximum utilization from multicore CPUs and modern GPUs.
Throughout these stages, timing and synchronization are paramount. Shared data structures—world transforms, physics snapshots—must be locked, double‐buffered, or designed lock‑free to prevent race conditions. Profiling tools highlight bottlenecks in input, physics, or rendering, guiding developers to balance workloads and maintain the loop’s target frame budget.
End of Loop: Building the Heartbeat of a Game
The game loop is far more than boilerplate; it underpins every interactive system, from input to audio to networking. A well‑architected loop delivers consistent pacing, bounded latency, and modular extensibility—allowing teams to plug in new features without disrupting the core heartbeat. As games grow in scope, loops evolve into sophisticated schedulers, prioritizing tasks by deadline and importance to maintain smooth simulation even under heavy loads.
In essence, mastering the game loop is mastering the engine itself. By carefully choosing update methods, ordering subsystems, and incorporating interpolation or multithreading, developers ensure that gameplay feels responsive, deterministic when needed, and visually seamless. The loop’s cadence defines the player’s tactile experience: it is the invisible metronome guiding every input, every collision, and every rendered frame.
Frequently Asked Questions
1. What is a game loop?
A game loop is the continuous cycle that processes input, updates game state, and renders frames, driving real‑time interactivity.
2. Why is delta time important?
Delta time (Δt) ensures frame‑rate–independent motion by scaling updates according to the actual time elapsed between frames.
3. When should I use fixed vs variable updates?
Use fixed updates for deterministic physics and multiplayer lockstep; variable updates for fluid responsiveness and higher frame rates.
4. How are input, physics, and rendering ordered?
Typically, loops poll input first, then update physics and game logic, and finally issue rendering commands to display the new frame.
5. How do I prevent frame‑rate spikes from breaking my game?
Clamp large Δt values, smooth frame‑time metrics, and consider hybrid loops (fixed physics, variable visuals) to avoid simulation instability.
Comments